📊 Reuse results using Metrics
Introduction
Metrics are a way to reuse previously calculated results in other statements. For instance, you can:
- Calculate the commission of a manager on the current period based on their team's performance
- Sum all the payouts of a sales rep at the end of year to perform a regulation
- Sum all the commissions paid to sales representative that involved with a specific customer over the last months
- Create an insight table in non-payout mode to show some KPIs of your team
- ... and pretty much anything that would need results from another statement.
What is indexed?
Currently we index:
- all statement variables that are defined in a KPI
- all statement variables that are used in a Total Rule
- all rule totals
- the total of the statement
- all field variables that have been marked as reusable metrics
null
value if it's not currently used in the statement. You still have to force its calculation, either by putting it as a KPI on a rule or by using it in a another formula.Creating a filter of metrics
Different aggregations
Before filtering metrics, you need to think of how you want the data to be structured, depending of your usecase.
- Statement Metric: you'll get the metrics aggregated by statement (one row per statement). You'll find all the metrics that have been indexed in the object, in addition to common keys of a statement (user, period, plan...). Useful to retrieve payouts or KPIs of other statements.
{
// Metadata from where the metric come from: statement, plan, period, user...
statementId: "...",
periodId: "...",
period: {id: "...", startDate: ...},
userId: "...",
user: {id: "...", email: ...},
planId: "...",
plan: {id: "...", name: "..."},
// Statement total
total: { value: 5000, symbol: "EUR" },
// Rules
rule_commissionBooking: { value: 1000, symbol: "EUR" },
// And extracted KPIs
targetReach: 0.688,
bookingMonth: { value: 4000, symbol: "EUR" },
}
- Object Metric: you'll get the metrics aggregated by statement and by row. Useful to reuse field variables of other statements.
[
{
// Metadata (see above)
...,
// Metadata about the current row
rowExternalId: "row1",
customObjectDefinitionMachineName: "opportunity",
// And extracted metrics from that row.
bookingCommission: { value: 5000, symbol: "EUR" },
commitmentPeriod: 12,
},
{
// Metadata (see above)
...,
// Metadata about the current row
rowExternalId: "row2",
customObjectDefinitionMachineName: "opportunity",
// And extracted metrics from that row.
bookingCommission: { value: 8000, symbol: 'EUR' },
commitmentPeriod: 36,
}
]
- Quota Metric: you'll get your list of quotas, useful to transform monthly quotas in yearly quotas or deduce team quotas based on individual ones.
[
{
// Infos about the user affected to the value.
userId: "...",
user: {id: "...", email: ...},
// Infos about the quota definition.
variableName: "target",
variableType: "currency",
// Period of validity and value.
startDate: "...",
endDate: "...",
value: 20000
}
]
Note that if you quickly need metrics reference in app, they're available in the Properties tab of the designer:
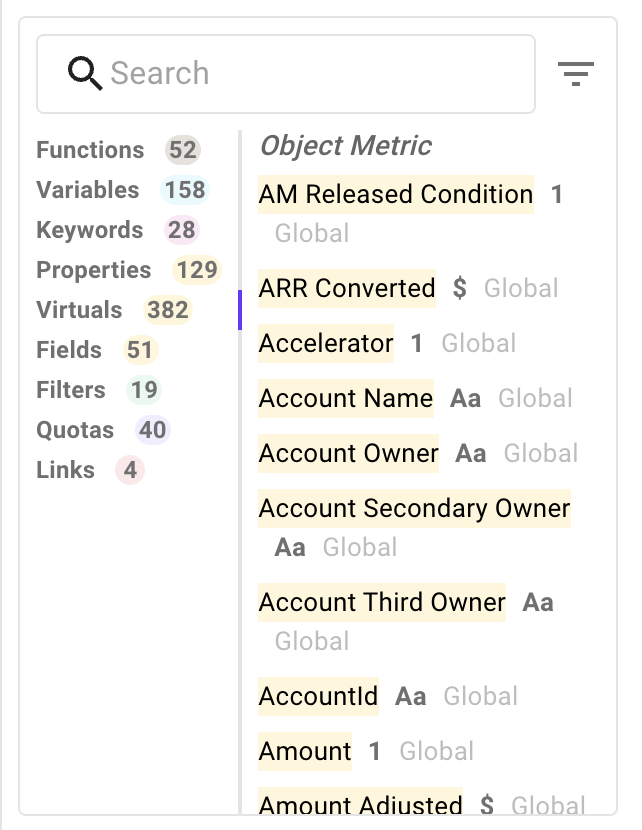
Writing filters to query your metrics
To get metrics, you can 📑 Create a Filter, but of type metrics, like so:
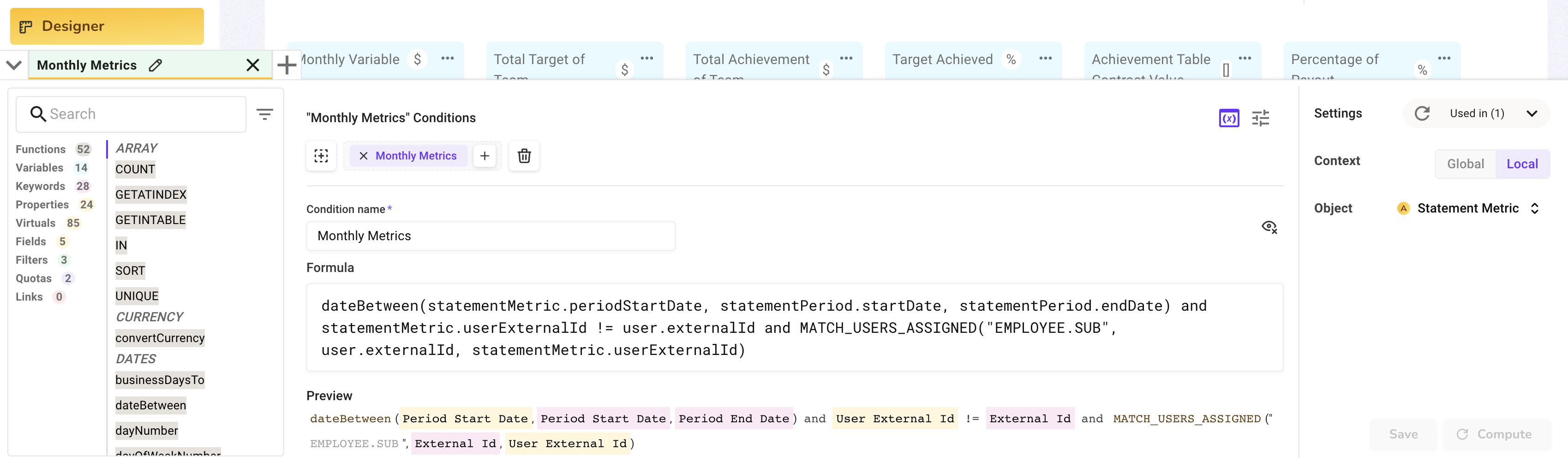
Examples:
MATCH(statementMetric.userExternalId, team.members, ["externalId"]) and isNotNull(statementMetric.bookingMonth) and statementMetric.periodId = statementPeriod.id
statementMetric.periodStartDate >= monthsAgo(statementPeriod.startDate,2) and statementMetric.periodEndDate < statementPeriod.startDate and statementMetric.userExternalId = user.externalId
statementMetric.periodStartDate >= monthsAgo(statementPeriod.startDate, 2) and statementMetric.periodEndDate < statementPeriod.startDate and statementMetric.userExternalId = user.externalId and statementMetric.plan.id = planAssignement.planId
First, you need to connect the opportunity to the object metrics that are associated. To do so, create a relation to link
opportunity
to the objectMetric
(let's call it previousPayments
) in 1 -> n:- Link all previous payments to this sales rep:
objectMetric.userExternalId = user.externalId and $row.externalId = objectMetric.rowExternalId
- Link all previous payments for periods previous to this statement:
objectMetric.userExternalId = user.externalId and $row.id = objectMetric.rowExternalId and objectMetric.periodStartDate <= statementPeriod.startDate
- Same but for last month only:
objectMetric.userExternalId = user.externalId and $row.id = objectMetric.rowExternalId and objectMetric.periodStartDate >= monthsAgo(statementPeriod.startDate, 1) and objectMetric.periodStartDate <= monthsAgo(statementPeriod.endDate, 1)
- You can now create an object variable on opportunity, for instance:
SUM(opportunity.previousPayments, objectMetric.bookingCommission)
- Create a filter to query all quota for the current user for the current year:
quota.user.externalId = user.externalId and quota.startDate >= statementPeriod.startOfYear and quota.endDate <= statementPeriod.endOfYear and quota.machineName = "target"
- Sum them all in a statement variable:
SUM(filter.quotaYear, quota.value)
Putting the filter on display
A metric filter is handled in the calculation engine as a regular filter, so you can put it on display using the plan configuration screen.
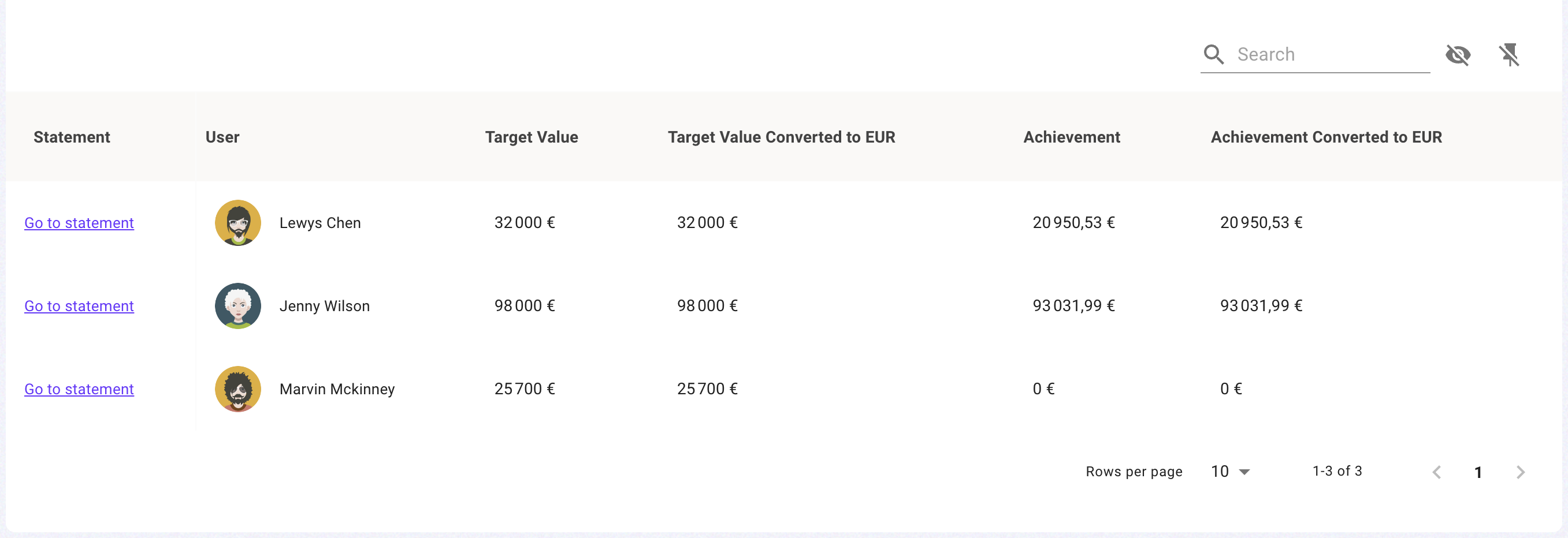
Using the values
The filter behaves like any others, you are now able to create statement variables and use common functions (SORT
, SUM
, GETATINDEX
...) like on any other filter, for example: SUM(filter.myTeamMembers, statementMetric.total)
. See examples above.